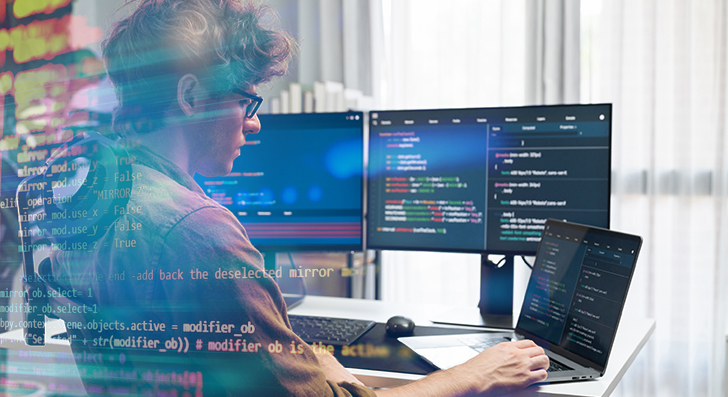
Scalability usually means your application can handle advancement—far more consumers, much more details, plus more targeted visitors—devoid of breaking. Like a developer, building with scalability in your mind will save time and anxiety afterwards. Below’s a clear and realistic guidebook to assist you start by Gustavo Woltmann.
Style and design for Scalability from the beginning
Scalability isn't a thing you bolt on later—it ought to be component within your program from the start. Several purposes are unsuccessful once they improve quickly for the reason that the initial structure can’t manage the additional load. As a developer, you need to Consider early regarding how your program will behave stressed.
Start by planning your architecture to be versatile. Prevent monolithic codebases exactly where everything is tightly linked. As a substitute, use modular design or microservices. These designs split your application into smaller, impartial sections. Each and every module or assistance can scale By itself with out impacting The full procedure.
Also, consider your database from day just one. Will it want to manage one million users or simply a hundred? Select the suitable style—relational or NoSQL—based on how your info will mature. Plan for sharding, indexing, and backups early, even if you don’t require them but.
One more significant issue is to avoid hardcoding assumptions. Don’t write code that only functions underneath latest disorders. Give thought to what would materialize When your consumer foundation doubled tomorrow. Would your app crash? Would the database slow down?
Use structure styles that support scaling, like information queues or party-pushed units. These enable your application tackle more requests with no finding overloaded.
Any time you Make with scalability in your mind, you're not just getting ready for success—you are decreasing long term complications. A very well-prepared technique is simpler to maintain, adapt, and grow. It’s improved to arrange early than to rebuild later on.
Use the correct Database
Picking out the appropriate database is really a vital Component of constructing scalable apps. Not all databases are constructed exactly the same, and utilizing the Completely wrong you can slow you down or simply bring about failures as your app grows.
Get started by knowledge your info. Can it be hugely structured, like rows inside a table? If Certainly, a relational database like PostgreSQL or MySQL is a superb in shape. They're strong with associations, transactions, and consistency. Additionally they support scaling approaches like read through replicas, indexing, and partitioning to handle additional site visitors and details.
Should your details is much more adaptable—like user action logs, product catalogs, or files—contemplate a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing big volumes of unstructured or semi-structured knowledge and can scale horizontally a lot more conveniently.
Also, contemplate your examine and publish styles. Are you currently executing lots of reads with less writes? Use caching and browse replicas. Are you presently handling a weighty generate load? Consider databases that could deal with substantial generate throughput, or even occasion-dependent details storage systems like Apache Kafka (for short-term knowledge streams).
It’s also clever to Imagine forward. You might not will need advanced scaling attributes now, but selecting a database that supports them signifies you gained’t will need to modify afterwards.
Use indexing to hurry up queries. Stay away from unneeded joins. Normalize or denormalize your facts based upon your obtain styles. And normally monitor databases performance as you increase.
In a nutshell, the right databases relies on your application’s composition, velocity desires, And just how you assume it to increase. Just take time to choose properly—it’ll help save a great deal of difficulties later on.
Optimize Code and Queries
Fast code is essential to scalability. As your application grows, every single modest delay adds up. Improperly written code or unoptimized queries can decelerate general performance and overload your process. That’s why it’s crucial that you Construct effective logic from the start.
Get started by producing clear, easy code. Avoid repeating logic and take away just about anything unwanted. Don’t select the most complicated Alternative if an easy a single works. Keep the features short, centered, and simple to check. Use profiling applications to seek out bottlenecks—locations where by your code normally takes as well extensive to run or uses an excessive amount memory.
Up coming, evaluate your database queries. These usually gradual items down more than the code by itself. Make sure Every single query only asks for the information you truly want. Stay clear of Pick *, which fetches everything, and alternatively pick unique fields. Use indexes to speed up lookups. And prevent doing too many joins, Primarily across massive tables.
If you recognize a similar information staying requested time and again, use caching. Store the outcomes briefly applying resources like Redis or Memcached and that means you don’t really have to repeat costly operations.
Also, batch your databases functions when you can. As opposed to updating a row one after the other, update them in groups. This cuts down on overhead and tends to make your app far more economical.
Make sure to test with big datasets. Code and queries that operate high-quality with a hundred documents might crash after they have to take care of 1 million.
In short, scalable apps are quickly apps. Maintain your code restricted, your read more queries lean, and use caching when essential. These methods enable your software continue to be sleek and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your app grows, it has to handle much more customers and even more site visitors. If almost everything goes by way of one particular server, it can rapidly become a bottleneck. That’s exactly where load balancing and caching come in. These two resources assist keep your application speedy, secure, and scalable.
Load balancing spreads incoming website traffic throughout several servers. As opposed to 1 server performing all the work, the load balancer routes buyers to different servers based on availability. This suggests no solitary server gets overloaded. If one server goes down, the load balancer can mail visitors to the Other individuals. Tools like Nginx, HAProxy, or cloud-centered alternatives from AWS and Google Cloud make this simple to setup.
Caching is about storing details briefly so it may be reused swiftly. When users ask for the identical info all over again—like a product page or maybe a profile—you don’t ought to fetch it in the databases each and every time. You can provide it in the cache.
There's two prevalent kinds of caching:
one. Server-side caching (like Redis or Memcached) suppliers info in memory for fast entry.
two. Consumer-facet caching (like browser caching or CDN caching) retailers static data files near to the person.
Caching minimizes databases load, enhances velocity, and helps make your application much more successful.
Use caching for things that don’t adjust often. And constantly make sure your cache is up-to-date when details does modify.
To put it briefly, load balancing and caching are easy but highly effective tools. Alongside one another, they help your app cope with more end users, continue to be quick, and Get well from complications. If you plan to expand, you require both.
Use Cloud and Container Equipment
To develop scalable purposes, you'll need applications that let your app increase quickly. That’s where cloud platforms and containers are available in. They provide you overall flexibility, lower set up time, and make scaling much smoother.
Cloud platforms like Amazon Internet Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and products and services as you need them. You don’t really need to obtain components or guess long run potential. When targeted visitors increases, you are able to include much more sources with only a few clicks or instantly making use of automobile-scaling. When site visitors drops, it is possible to scale down to save cash.
These platforms also supply expert services like managed databases, storage, load balancing, and protection instruments. You may center on constructing your app as opposed to handling infrastructure.
Containers are An additional key Software. A container offers your application and every little thing it must run—code, libraries, configurations—into one particular unit. This causes it to be straightforward to move your application amongst environments, out of your laptop into the cloud, devoid of surprises. Docker is the most well-liked tool for this.
Once your app utilizes multiple containers, instruments like Kubernetes allow you to handle them. Kubernetes handles deployment, scaling, and Restoration. If just one element of the app crashes, it restarts it quickly.
Containers also ensure it is easy to different areas of your application into companies. You are able to update or scale pieces independently, that's great for functionality and reliability.
Briefly, utilizing cloud and container applications implies you could scale rapidly, deploy easily, and Get better swiftly when complications take place. If you want your app to mature without having restrictions, begin working with these tools early. They preserve time, cut down danger, and make it easier to stay focused on making, not fixing.
Check All the things
In the event you don’t observe your application, you gained’t know when points go wrong. Monitoring will help the thing is how your application is carrying out, place troubles early, and make improved decisions as your app grows. It’s a essential Element of building scalable techniques.
Start off by monitoring essential metrics like CPU use, memory, disk space, and reaction time. These inform you how your servers and products and services are doing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you acquire and visualize this facts.
Don’t just observe your servers—monitor your app too. Keep an eye on how long it will take for consumers to load webpages, how often problems take place, and the place they arise. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring inside your code.
Arrange alerts for vital complications. Such as, In the event your reaction time goes earlier mentioned a Restrict or possibly a provider goes down, you must get notified right away. This aids you repair problems fast, often right before people even observe.
Monitoring can also be useful after you make improvements. In case you deploy a completely new element and see a spike in errors or slowdowns, you could roll it back again just before it causes serious hurt.
As your app grows, targeted visitors and facts boost. Without checking, you’ll skip indications of difficulties till it’s much too late. But with the best resources set up, you remain on top of things.
In a nutshell, checking will help you keep your application trustworthy and scalable. It’s not pretty much spotting failures—it’s about understanding your technique and making sure it really works well, even stressed.
Final Feelings
Scalability isn’t only for huge providers. Even tiny applications want a solid foundation. By coming up with carefully, optimizing correctly, and utilizing the proper instruments, you are able to Make apps that expand effortlessly with out breaking stressed. Commence smaller, think massive, and Establish intelligent.