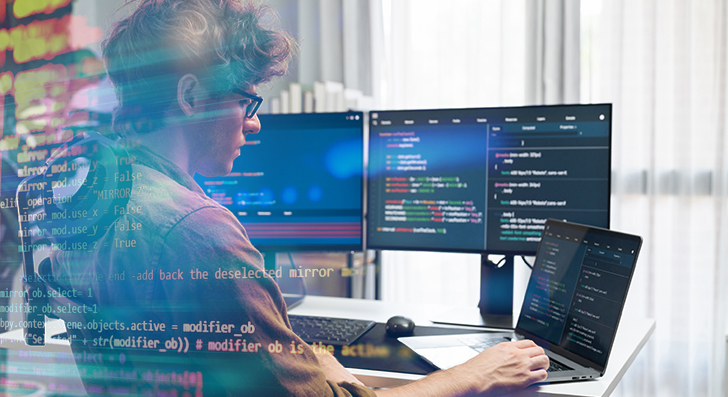
Scalability implies your application can manage development—more buyers, far more info, and much more traffic—without the need of breaking. For a developer, creating with scalability in your mind will save time and tension afterwards. Listed here’s a transparent and realistic guidebook to help you start out by Gustavo Woltmann.
Design and style for Scalability from the beginning
Scalability isn't really some thing you bolt on later on—it should be aspect of one's system from the beginning. Several purposes fall short every time they expand fast due to the fact the original layout can’t handle the extra load. As being a developer, you might want to Feel early regarding how your system will behave under pressure.
Get started by developing your architecture being flexible. Stay clear of monolithic codebases in which all the things is tightly connected. Alternatively, use modular structure or microservices. These patterns split your application into lesser, independent pieces. Each and every module or provider can scale By itself with out impacting The full procedure.
Also, consider your database from day one. Will it require to deal with 1,000,000 people or just a hundred? Choose the proper form—relational or NoSQL—dependant on how your info will increase. Approach for sharding, indexing, and backups early, Even when you don’t need them however.
Yet another critical place is to stop hardcoding assumptions. Don’t generate code that only functions beneath recent problems. Contemplate what would transpire In the event your person foundation doubled tomorrow. Would your application crash? Would the database decelerate?
Use style and design styles that guidance scaling, like concept queues or function-driven techniques. These aid your app deal with far more requests with no receiving overloaded.
If you Create with scalability in mind, you're not just making ready for fulfillment—you happen to be minimizing future headaches. A very well-planned procedure is less complicated to keep up, adapt, and develop. It’s much better to prepare early than to rebuild later on.
Use the appropriate Database
Choosing the right databases can be a crucial Portion of developing scalable purposes. Not all databases are constructed exactly the same, and utilizing the Mistaken one can slow you down or simply lead to failures as your app grows.
Get started by being familiar with your knowledge. Could it be very structured, like rows in a desk? If yes, a relational databases like PostgreSQL or MySQL is an efficient match. These are definitely sturdy with relationships, transactions, and regularity. Additionally they help scaling techniques like examine replicas, indexing, and partitioning to deal with more targeted visitors and knowledge.
Should your data is a lot more adaptable—like consumer activity logs, product or service catalogs, or documents—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at handling massive volumes of unstructured or semi-structured details and may scale horizontally extra simply.
Also, think about your read through and write patterns. Will you be performing a great deal of reads with much less writes? Use caching and read replicas. Have you been managing a weighty generate load? Consider databases that could tackle higher publish throughput, or maybe event-primarily based knowledge storage units like Apache Kafka (for short term facts streams).
It’s also smart to Believe forward. You may not need to have Highly developed scaling features now, but choosing a database that supports them implies you gained’t have to have to switch later.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your knowledge determined by your obtain styles. And normally observe databases efficiency while you expand.
In a nutshell, the best database is determined by your app’s construction, speed requirements, and how you anticipate it to increase. Just take time to choose properly—it’ll save a lot of trouble afterwards.
Improve Code and Queries
Rapid code is vital to scalability. As your app grows, each modest delay adds up. Improperly published code or unoptimized queries can slow down efficiency and overload your method. That’s why it’s crucial to build economical logic from the start.
Begin by writing thoroughly clean, simple code. Stay clear of repeating logic and take away anything at all pointless. Don’t pick the most intricate Remedy if a simple just one performs. Keep your functions small, targeted, and easy to check. Use profiling resources to uncover bottlenecks—spots exactly where your code usually takes way too lengthy to operate or makes use of a lot of memory.
Next, check out your database queries. These generally slow points down over the code alone. Ensure Each individual query only asks for the info you actually will need. Prevent Choose *, which fetches anything, and rather pick out particular fields. Use indexes to hurry up lookups. And stay clear of carrying out a lot of joins, Particularly throughout significant tables.
Should you discover precisely the same data getting asked for many times, use caching. Retailer the final results quickly utilizing equipment like Redis or Memcached this means you don’t need to repeat high-priced functions.
Also, batch your database operations if you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and can make your application extra efficient.
Remember to examination with massive datasets. Code and queries that do the job fine with one hundred data could crash when they have to handle 1 million.
In brief, scalable apps are quickly apps. Maintain your code restricted, your queries lean, and use caching when essential. These methods enable your application stay smooth and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to handle more customers and much more site visitors. If every little thing goes by way of one particular server, it is going to immediately turn into a bottleneck. That’s wherever load balancing and caching can be found in. Both of these equipment aid keep your app speedy, secure, and scalable.
Load balancing spreads incoming website traffic throughout several servers. As opposed to 1 server undertaking each of the perform, the load balancer routes customers to different servers dependant on availability. What this means is no solitary server gets overloaded. If a person server goes down, the load balancer can send out visitors to the Other people. Tools like Nginx, HAProxy, or cloud-centered remedies from AWS and Google Cloud make this very easy to create.
Caching is about click here storing information quickly so it could be reused rapidly. When users ask for the identical information yet again—like a product page or maybe a profile—you don’t must fetch it from the databases whenever. You are able to provide it from your cache.
There's two widespread kinds of caching:
one. Server-side caching (like Redis or Memcached) outlets information in memory for speedy accessibility.
two. Consumer-facet caching (like browser caching or CDN caching) shops static files near to the user.
Caching lowers databases load, enhances velocity, and tends to make your application much more successful.
Use caching for things that don’t adjust normally. And often be certain your cache is up to date when facts does adjust.
In short, load balancing and caching are straightforward but impressive resources. Jointly, they help your app take care of extra consumers, keep fast, and Recuperate from troubles. If you propose to develop, you may need each.
Use Cloud and Container Equipment
To develop scalable purposes, you'll need equipment that allow your application mature effortlessly. That’s in which cloud platforms and containers can be found in. They offer you adaptability, reduce setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World wide web Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you require them. You don’t really have to buy hardware or guess future capacity. When visitors raises, you'll be able to incorporate far more methods with just a couple clicks or mechanically working with automobile-scaling. When site visitors drops, you'll be able to scale down to save cash.
These platforms also provide providers like managed databases, storage, load balancing, and safety resources. You'll be able to give attention to developing your app instead of running infrastructure.
Containers are A further critical Resource. A container deals your app and every little thing it must run—code, libraries, configurations—into one particular unit. This makes it quick to maneuver your app between environments, from a laptop computer for the cloud, with out surprises. Docker is the preferred Resource for this.
Whenever your app works by using a number of containers, instruments like Kubernetes enable you to handle them. Kubernetes handles deployment, scaling, and Restoration. If one particular component within your app crashes, it restarts it immediately.
Containers also enable it to be very easy to separate portions of your app into products and services. It is possible to update or scale components independently, which happens to be great for performance and dependability.
In short, using cloud and container instruments indicates you may scale quickly, deploy conveniently, and Recuperate immediately when difficulties materialize. If you need your application to expand without the need of limitations, start out utilizing these equipment early. They save time, minimize hazard, and enable you to stay focused on making, not correcting.
Check Anything
If you don’t keep an eye on your software, you received’t know when issues go Mistaken. Checking helps you see how your app is undertaking, location problems early, and make greater conclusions as your application grows. It’s a key Portion of setting up scalable methods.
Commence by monitoring primary metrics like CPU use, memory, disk space, and response time. These let you know how your servers and companies are executing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you collect and visualize this info.
Don’t just keep an eye on your servers—keep an eye on your application far too. Regulate how much time it's going to take for users to load pages, how often mistakes take place, and the place they arise. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Put in place alerts for critical troubles. One example is, If the reaction time goes previously mentioned a limit or perhaps a services goes down, you need to get notified instantly. This assists you fix issues speedy, normally in advance of end users even see.
Checking can be beneficial whenever you make changes. For those who deploy a different attribute and see a spike in errors or slowdowns, you may roll it back again prior to it causes authentic hurt.
As your app grows, targeted visitors and knowledge boost. Without checking, you’ll skip indications of difficulties till it’s much too late. But with the ideal instruments in place, you keep in control.
To put it briefly, monitoring allows you maintain your application reputable and scalable. It’s not just about recognizing failures—it’s about comprehending your process and ensuring it really works nicely, even stressed.
Last Feelings
Scalability isn’t just for massive companies. Even modest apps need to have a strong foundation. By building very carefully, optimizing sensibly, and using the ideal resources, you could Develop applications that grow easily devoid of breaking under pressure. Commence compact, Believe major, and build wise.